Operator screen to control 4 variables at a given coordinate on a grid.
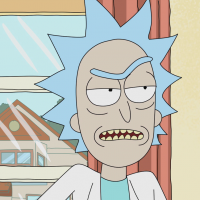
We want to be able to set a single point in the center of a grid.
Using that center point, we use offsets from there to get the positions we want.
The program data we want operators to be able to access for a given point on the grid is:
· Timing of spray head
· Pattern of spray head
· Possible waittime
· A Boolean to determine if we use the position or not (used for the screen maker later on)
-36in to 36in with a resolution of 0.5in for two axes creates 20736 routines and 82944 variables.
Ideally, while the robot is running, an operator can press a grid point (picture of grid) with the pendant. This gives them opportunity to change one of four variables.
Is there a more compact way to code this?
Is it necessary to create this many variables, in order to store everything for later use?
Ex:
CONST robtarget centerpos:=[[-490.16,1517.62,1804.67],[0.262408,-0.265629,-0.646938,0.664872],[1,0,-1,1],[9E+09,9E+09,9E+09,9E+09,9E+09,9E+09]];
PERS num row:=0
PERS num column:=0
PERS num timing0_0.25:=0
PERS num pattern0_0.25:=0
PERS num waittime0_0.25:=0
PERS bool positionused0_0.25:=FALSE
PERS num timing0_0.5:=0
PERS num pattern0_0.5:=0
PERS num waittime0_0.5:=0
PERS bool positionused0_0.5:=FALSE
PERS num timing0_0.75:=0
PERS num pattern0_0.75:=0
PERS num waittime0_0.75:=0
PERS bool positionused0_0.75:=FALSE
PERS num timing0_1:=0
PERS num pattern0_1:=0
PERS num waittime0_1:=0
PERS bool positionused0_1:=FALSE
Proc 0,0.25()
row:=0
column:=0.25
spraypos:=Offs(centerpos, row*6.35, column*6.35, 0);
MoveL spraypos, v400, fine, t_spray;
timing0_0.25:=1
pattern0_0.25:=5
waittime0_0.25:=0
positionused0_0.25:=TRUE
Return;
EndProc
Proc 0,0.5()
row:=0
column:=0.5
spraypos:=Offs(centerpos, row*6.35, column*6.35, 0);
MoveL spraypos, v400, fine, t_spray;
timing0_0.5:=2
pattern0_0.5:=6
waittime0_0.25:=0
positionused0_0.5:=TRUE
Return;
EndProc
Proc 0,0.75()
row:=0
column:=0.75
spraypos:=Offs(centerpos, row*6.35, column*6.35, 0);
timing0_0.75:=2
pattern0_0.75:=6
waittime0_0.75:=0
positionused0_0.75:=TRUE
Return;
EndProc
Proc 0,1()
row:=0
column:=1
spraypos:=Offs(centerpos, row*6.35, column*6.35, 0);
timing0_1:=2
pattern0_1:=6
waittime0_1:=0
positionused0_1:=TRUE
Return;
EndProc
PROC Spray_Path()
MoveL Home, v400, z50, t_spray;
MoveL above_die, v400, z50, t_spray;
0,0.75;
0,1;
0,0.25;
0,1;
0,0.5;
MoveL above_die, v400, z50, t_spray;
MoveL Home, v400, z50, t_spray;
EndProc
Categories
- All Categories
- 5.6K RobotStudio
- 400 UpFeed
- 20 Tutorials
- 14 RobotApps
- 301 PowerPacs
- 406 RobotStudio S4
- 1.8K Developer Tools
- 250 ScreenMaker
- 2.8K Robot Controller
- 348 IRC5
- 74 OmniCore
- 8 RCS (Realistic Controller Simulation)
- 845 RAPID Programming
- 21 AppStudio
- 4 RobotStudio AR Viewer
- 19 Wizard Easy Programming
- 109 Collaborative Robots
- 5 Job listings