PC SDK EventLog Domain

bgrant
✭
Hi all,
I have an application that was working fine but is now throwing an exception on null reference. I am working with the event log domain. I have highlighted the line of code that throws the exception below. Thanks in advance!
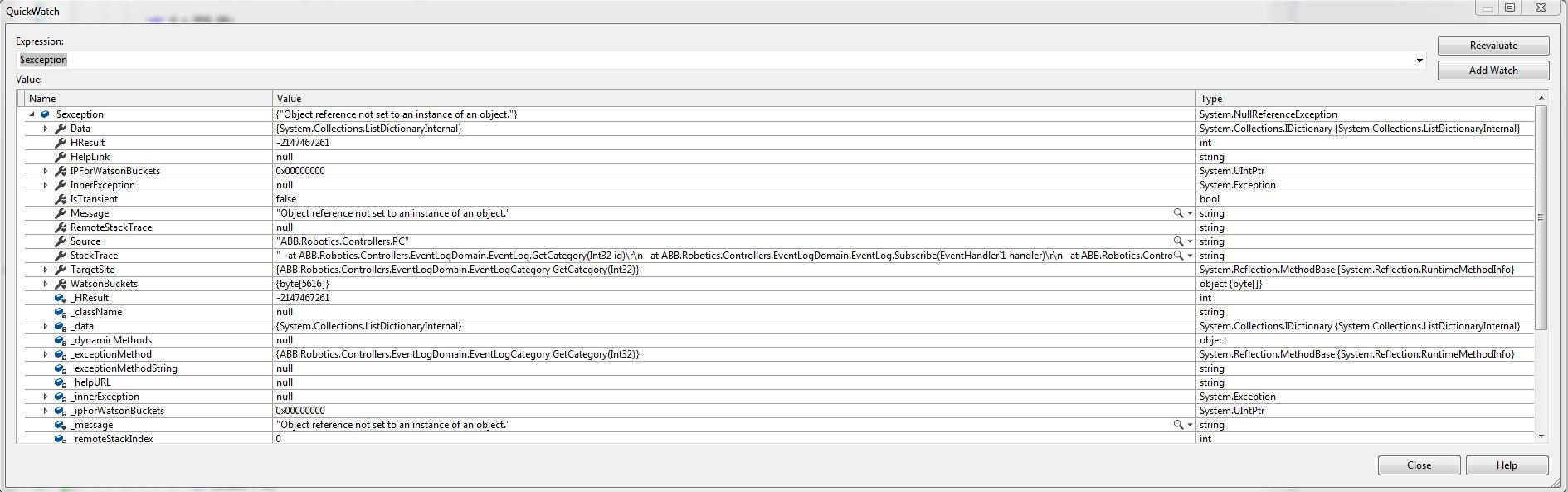
I have an application that was working fine but is now throwing an exception on null reference. I am working with the event log domain. I have highlighted the line of code that throws the exception below. Thanks in advance!
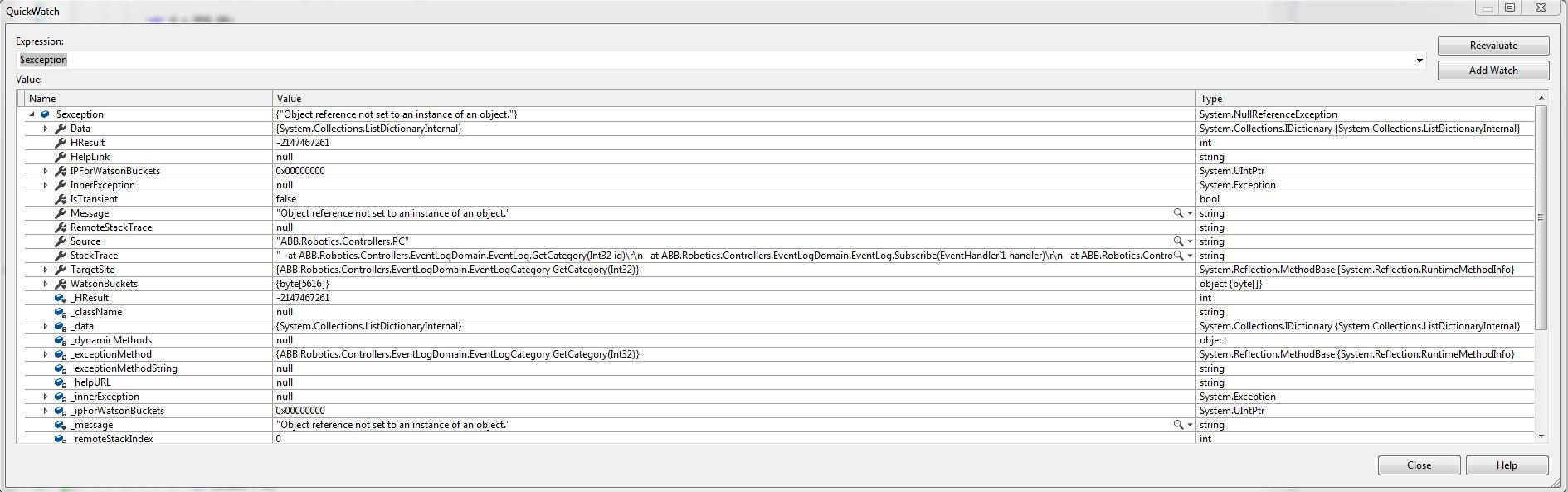
using System;
using System.Text;
using System.Data.SqlClient;
using ABB.Robotics.Controllers;
using ABB.Robotics.Controllers.Messaging;
using ABB.Robotics.Controllers.Discovery;
using ABB.Robotics.Controllers.EventLogDomain;
namespace ControllerAPI
{
class Program
{
static string GUID = System.Configuration.ConfigurationManager.AppSettings["System_ID"];
static string RMQ_QN = System.Configuration.ConfigurationManager.AppSettings["RMQ_Queue_Name"];
static string RMQ_SQL_SN = System.Configuration.ConfigurationManager.AppSettings["RMQ_SQL_Server_Name"];
static string RMQ_SQL_DBN = System.Configuration.ConfigurationManager.AppSettings["RMQ_SQL_DB_Name"];
static string RMQ_SQL_UN = System.Configuration.ConfigurationManager.AppSettings["RMQ_SQL_Username"];
static string RMQ_SQL_PW = System.Configuration.ConfigurationManager.AppSettings["RMQ_SQL_Password"];
static string RMQ_SQL_SP = System.Configuration.ConfigurationManager.AppSettings["RMQ_SQL_Stored_Proc"];
static string EL_SQL_SN = System.Configuration.ConfigurationManager.AppSettings["EL_SQL_Server_Name"];
static string EL_SQL_DBN = System.Configuration.ConfigurationManager.AppSettings["EL_SQL_DB_Name"];
static string EL_SQL_UN = System.Configuration.ConfigurationManager.AppSettings["EL_SQL_Username"];
static string EL_SQL_PW = System.Configuration.ConfigurationManager.AppSettings["EL_SQL_Password"];
static string EL_SQL_SP = System.Configuration.ConfigurationManager.AppSettings["EL_SQL_Stored_Proc"];
/// <summary>
/// The main entry point for the application.
/// </summary>
[MTAThread]
static void Main(string[] args)
{
NetworkScanner scanner = new NetworkScanner();
scanner.Scan();
// Wait a while for the scan to find more controllers if needed.
System.Threading.Thread.Sleep(2000);
scanner.Scan();
IpcQueue myQueue;
IpcMessage recMessage;
Controller ctrl;
ctrl = CreateController();
ctrl.Logon(UserInfo.DefaultUser);
Console.WriteLine("Controller-System: {0} time is: {1} System ID is: {2}", ctrl.SystemName, ctrl.DateTime, ctrl.SystemId);
Console.WriteLine("Press any key to terminate");
var Q = RMQ_QN;
string msg = "";
int index = 0;
int queueid = (ctrl.Ipc.GetQueueId(Q));
if (ctrl.Ipc.Exists(Q))
{
ctrl.Ipc.DeleteQueue(queueid);
myQueue = ctrl.Ipc.CreateQueue(Q, 2, Ipc.IPC_MAXMSGSIZE);
}
else
{
myQueue = ctrl.Ipc.CreateQueue(Q, 2, Ipc.IPC_MAXMSGSIZE);
}
myQueue = ctrl.Ipc.GetQueue(Q);
while (true)
{
ctrl.EventLog.MessageWritten += EventLogMessageWritten;
recMessage = (new IpcMessage());
IpcReturnType ret = IpcReturnType.Timeout;
string answer = string.Empty;
int timeout = 5000;
//Check for msg in the PC SDK queue
ret = myQueue.Receive(timeout, recMessage);
if (ret == IpcReturnType.OK)
{
//convert msg data to string
answer = new UTF8Encoding().GetString(recMessage.Data);
msg = answer.Trim(' ');
msg = msg.Remove(0, 8);
index = msg.LastIndexOf(">");
if (index > 0)
{
msg = msg.Substring(0, index + 1);
Console.WriteLine(msg.Length.ToString());
Console.WriteLine(msg);
LogMessage(msg);
}
}
else
{
Console.WriteLine("Timeout!");
}
}
}
static Controller CreateController()
{
try
{
NetworkScanner scanner = new NetworkScanner();
Controller @static = new Controller(ControllerFactory.FormatControllerId(new Guid(GUID)));
return @static;
}
catch (Exception ex)
{
Console.WriteLine("The specified GUID cannot be found. The robot GUID can be found in the internal directory in the 'system.guid' file. The current sysID is: " + GUID);
Console.WriteLine("Press any key to terminate");
Console.ReadKey();
Environment.Exit(1);
}
return null;
}
public static void LogMessage(object msg)
{
using (SqlConnection connection = new SqlConnection("Data Source=" + RMQ_SQL_SN + ";Initial Catalog=" + RMQ_SQL_DBN + ";User Id=" + RMQ_SQL_UN + ";Password=" + RMQ_SQL_PW))
{
SqlCommand command = new SqlCommand(RMQ_SQL_SP, connection);
command.CommandType = System.Data.CommandType.StoredProcedure;
// make a sqlparameter object that we'll use for each param
SqlParameter sp;
string xml = "<BODY>" + "<GUID>" + GUID + "</GUID>" + msg + "</BODY>";
sp = new SqlParameter("@xml", System.Data.SqlDbType.Xml);
sp.SqlValue = xml;
command.Parameters.Add(sp);
connection.Open();
command.ExecuteNonQuery();
}
}
public static void EventLogMessageWritten(object sender, MessageWrittenEventArgs e)
{
if (sender != null)
if (e != null)
{
Console.WriteLine(e.Message.Title);
//Console.WriteLine("EVENT HAPPENED");
//using (SqlConnection connection = new SqlConnection("Data Source=;Initial Catalog=;User Id=;Password=
// string query = "INSERT into dbo.ABBEventLog (Data) VALUES (@A)";
// using (SqlCommand command = new SqlCommand(query, connection))
// {
// command.Parameters.AddWithValue("@A", "<A>" + "<SYSID>" + GUID + "</SYSID>" + "<TYPE>" + e.Message.Type + "</TYPE>" + "<CATEGORY>" + e.Message.CategoryId + "</CATEGORY>" + "<MNUM>" + e.Message.SequenceNumber + "</MNUM>" + "<TIME>" + e.Message.Timestamp + "</TIME>" + e.Message.Body + "</A>");
// connection.Open();
// command.ExecuteNonQuery();
// }
// }
}
else
Console.WriteLine("Null Event Log Message");
}
}
}
0
Comments
-
UPDATE:
I have tried this code with a virtual controller running in RobotStudio 6.08.8307.1040 and I can write any attribute of the event log message to the console. Could my issue be that I have to use the version of ABB.Robotics.Controllers.PC.dll that corresponds to my real controller RobotWare version? I am currently using ABB.Robotics.Controllers.PC.dll from PC SDK 6.08.0
Categories
- All Categories
- 5.6K RobotStudio
- 399 UpFeed
- 20 Tutorials
- 14 RobotApps
- 301 PowerPacs
- 406 RobotStudio S4
- 1.8K Developer Tools
- 250 ScreenMaker
- 2.8K Robot Controller
- 341 IRC5
- 68 OmniCore
- 8 RCS (Realistic Controller Simulation)
- 839 RAPID Programming
- 18 AppStudio
- 4 RobotStudio AR Viewer
- 19 Wizard Easy Programming
- 107 Collaborative Robots
- 5 Job listings