Why are all the urls I filled in my subscription invalid?What format should it be?
Options
Sample
✭
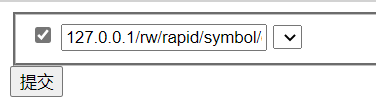
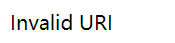
The above is the value in the address box below, but the invalid URI is displayed when the form is submitted

If it's typed directly into the url box there's no problem, how do you solve that problem?
Tagged:
0
Best Answer
-
Here is an example code of subscription using Python 3. Take a look at the formatting of the URLs. I hope it can give you some guidance.
import sys, argparse <br> import xml.etree.ElementTree as ET <br> from ws4py.client.threadedclient import WebSocketClient <br> import requests <br> import json <br> from requests.auth import HTTPDigestAuth <br> <br> namespace = '{http://www.w3.org/1999/xhtml}' <br> <br> def print_event(evt): <br> root = ET.fromstring(evt) <br> if root.findall(".//{0}li[@class='pnl-ctrlstate-ev']".format(namespace)): <br> print ("\tController State : " + root.find(".//{0}li[@class='pnl-ctrlstate-ev']/{0}span".format(namespace)).text) <br> if root.findall(".//{0}li[@class='pnl-opmode-ev']".format(namespace)): <br> print ("\tOperation Mode : " + root.find(".//{0}li[@class='pnl-opmode-ev']/{0}span".format(namespace)).text) <br> if root.findall(".//{0}li[@class='pnl-speedratio-ev']".format(namespace)): <br> print ("\tSpeed Ratio : " + root.find(".//{0}li[@class='pnl-speedratio-ev']/{0}span".format(namespace)).text) <br> <br> class RobWebSocketClient(WebSocketClient): <br> def opened(self): <br> print ("Web Sockect connection established") <br> <br> def closed(self, code, reason=None): <br> print ("Closed down", code, reason) <br> <br> def received_message(self, event_xml): <br> if event_xml.is_text: <br> print ("Events : ") <br> print_event(event_xml.data.decode("utf-8")) <br> else: <br> print ("Received Illegal Event " + str(event_xml)) <br> <br> class RWPanel: <br> def __init__(self, host, username, password): <br> self.host = host <br> self.username = username <br> self.password = password <br> self.digest_auth = HTTPDigestAuth(self.username,self.password) <br> self.subscription_url = 'http://{0}/subscription'.format(self.host) <br> self.session = requests.Session() <br> <br> def subscribe(self): <br> payload = {'resources':['1','2','3'], '1':'/rw/panel/speedratio', '1-p':'1', '2':'/rw/panel/ctrlstate', '2-p':'1', '3':'/rw/panel/opmode', '3-p':'1'} <br> <br> resp = self.session.post(self.subscription_url , auth=self.digest_auth, data=payload) <br> print ("Initial Events : ") <br> print_event(resp.text) <br> if resp.status_code == 201: <br> self.location = resp.headers['Location'] <br> self.cookie = '-http-session-={0}; ABBCX={1}'.format(resp.cookies['-http-session-'], resp.cookies['ABBCX']) <br> return True <br> else: <br> print ('Error subscribing ' + str(resp.status_code)) <br> return False <br> <br> def start_recv_events(self): <br> self.header = [('Cookie',self.cookie)] <br> self.ws = RobWebSocketClient(self.location, protocols=['robapi2_subscription'], headers=self.header) <br> self.ws.connect() <br> self.ws.run_forever() <br> <br> def close(self): <br> self.ws.close() <br> def main(): <br> try: <br> parser = argparse.ArgumentParser() <br> parser.add_argument("-host",help="The host to connect. Defaults to localhost on port 80", default='localhost:80') <br> parser.add_argument("-user",help="The login user name. Defaults to default user name", default='Default User') <br> parser.add_argument("-passcode",help="The login password. Defaults to default password", default='robotics') <br> <br> if rwpanel.subscribe(): <br> rwpanel.start_recv_events() <br> <br> except KeyboardInterrupt: <br> rwpanel.close() <br> <br> if __name__ == "__main__": <br> main(sys.argv[1:])
5
Answers
-
dnilsson said:Here is an example code of subscription using Python 3. Take a look at the formatting of the URLs. I hope it can give you some guidance.
import sys, argparse <br> import xml.etree.ElementTree as ET <br> from ws4py.client.threadedclient import WebSocketClient <br> import requests <br> import json <br> from requests.auth import HTTPDigestAuth <br> <br> namespace = '{http://www.w3.org/1999/xhtml}' <br> <br> def print_event(evt): <br> root = ET.fromstring(evt) <br> if root.findall(".//{0}li[@class='pnl-ctrlstate-ev']".format(namespace)): <br> print ("\tController State : " + root.find(".//{0}li[@class='pnl-ctrlstate-ev']/{0}span".format(namespace)).text) <br> if root.findall(".//{0}li[@class='pnl-opmode-ev']".format(namespace)): <br> print ("\tOperation Mode : " + root.find(".//{0}li[@class='pnl-opmode-ev']/{0}span".format(namespace)).text) <br> if root.findall(".//{0}li[@class='pnl-speedratio-ev']".format(namespace)): <br> print ("\tSpeed Ratio : " + root.find(".//{0}li[@class='pnl-speedratio-ev']/{0}span".format(namespace)).text) <br> <br> class RobWebSocketClient(WebSocketClient): <br> def opened(self): <br> print ("Web Sockect connection established") <br> <br> def closed(self, code, reason=None): <br> print ("Closed down", code, reason) <br> <br> def received_message(self, event_xml): <br> if event_xml.is_text: <br> print ("Events : ") <br> print_event(event_xml.data.decode("utf-8")) <br> else: <br> print ("Received Illegal Event " + str(event_xml)) <br> <br> class RWPanel: <br> def __init__(self, host, username, password): <br> self.host = host <br> self.username = username <br> self.password = password <br> self.digest_auth = HTTPDigestAuth(self.username,self.password) <br> self.subscription_url = 'http://{0}/subscription'.format(self.host) <br> self.session = requests.Session() <br> <br> def subscribe(self): <br> payload = {'resources':['1','2','3'], '1':'/rw/panel/speedratio', '1-p':'1', '2':'/rw/panel/ctrlstate', '2-p':'1', '3':'/rw/panel/opmode', '3-p':'1'} <br> <br> resp = self.session.post(self.subscription_url , auth=self.digest_auth, data=payload) <br> print ("Initial Events : ") <br> print_event(resp.text) <br> if resp.status_code == 201: <br> self.location = resp.headers['Location'] <br> self.cookie = '-http-session-={0}; ABBCX={1}'.format(resp.cookies['-http-session-'], resp.cookies['ABBCX']) <br> return True <br> else: <br> print ('Error subscribing ' + str(resp.status_code)) <br> return False <br> <br> def start_recv_events(self): <br> self.header = [('Cookie',self.cookie)] <br> self.ws = RobWebSocketClient(self.location, protocols=['robapi2_subscription'], headers=self.header) <br> self.ws.connect() <br> self.ws.run_forever() <br> <br> def close(self): <br> self.ws.close() <br> def main(): <br> try: <br> parser = argparse.ArgumentParser() <br> parser.add_argument("-host",help="The host to connect. Defaults to localhost on port 80", default='localhost:80') <br> parser.add_argument("-user",help="The login user name. Defaults to default user name", default='Default User') <br> parser.add_argument("-passcode",help="The login password. Defaults to default password", default='robotics') <br> <br> if rwpanel.subscribe(): <br> rwpanel.start_recv_events() <br> <br> except KeyboardInterrupt: <br> rwpanel.close() <br> <br> if __name__ == "__main__": <br> main(sys.argv[1:])
0
Categories
- All Categories
- 5.6K RobotStudio
- 399 UpFeed
- 20 Tutorials
- 14 RobotApps
- 301 PowerPacs
- 406 RobotStudio S4
- 1.8K Developer Tools
- 250 ScreenMaker
- 2.8K Robot Controller
- 345 IRC5
- 73 OmniCore
- 8 RCS (Realistic Controller Simulation)
- 843 RAPID Programming
- 20 AppStudio
- 4 RobotStudio AR Viewer
- 19 Wizard Easy Programming
- 107 Collaborative Robots
- 5 Job listings