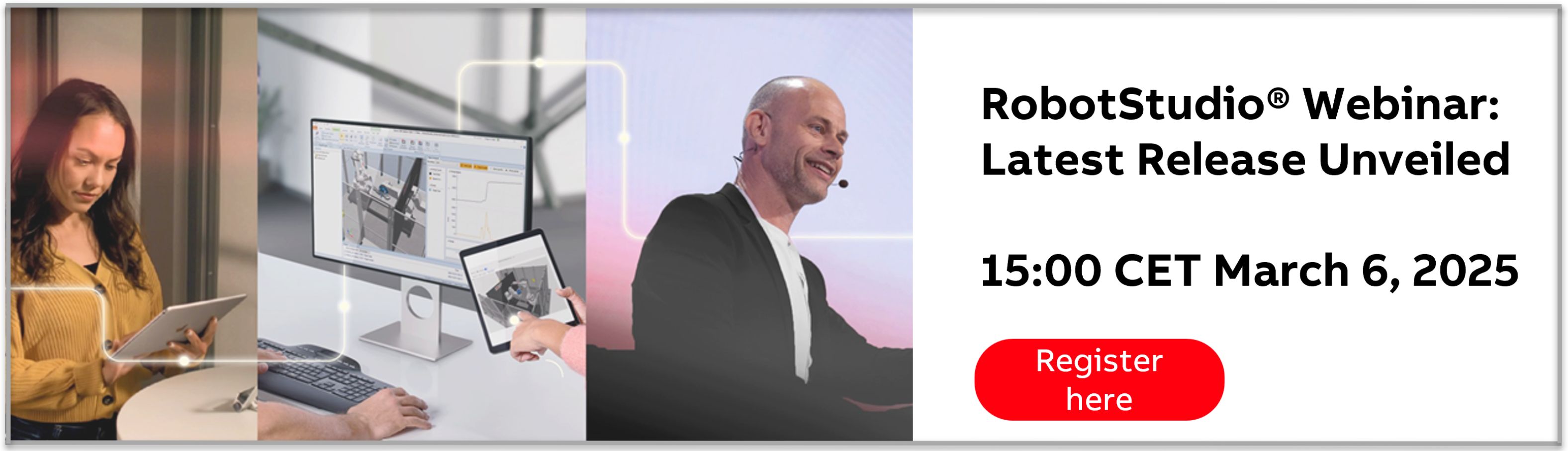
API: Create and attach tool from geometry

Matthias
✭
in RobotStudio
Hi,
I am trying to create a tool from a geometry.
There is an Xml-file with the data I need for the tool.
It stores the path to the Cad-file, its center of gravity, its position in the wrist coordinate system and so on.
I can import the geometry from a given CAD-model via
[code]
GraphicComponentLibrary gcl = GraphicComponentLibrary.Load(reader.GetAttribute("path"), false);
station.GraphicComponents.Add(gcl.RootComponent.CopyInstance ());
[/code]
I can also create a tool with data from an Xml-reader via
[code]
RsToolData tool = new RsToolData();
tool.ModuleName = "Module1";
tool.Name = reader.GetAttribute("name");
tool.RobotHold = true;
tool.Frame.X = Convert.ToDouble(reader.GetAttribute("x"));
tool.Frame.Y = Convert.ToDouble(reader.GetAttribute("y"));
tool.Frame.Z = Convert.ToDouble(reader.GetAttribute("z"));
tool.Frame.RX = Globals.DegToRad(Convert.ToDouble(reader.GetAttribute("rx")) );
tool.Frame.RY = Globals.DegToRad(Convert.ToDouble(reader.GetAttribute("ry")) );
tool.Frame.RZ = Globals.DegToRad(Convert.ToDouble(reader.GetAttribute("rz")) );
//Create the loaddata for the tool
RsLoadData myloadData = new RsLoadData();
//Set mass
myloadData.Mass = Convert.ToDouble(reader.GetAttribute("mass"));
//Set center of gravity
myloadData.Cog = new Vector3(Convert.ToDouble(reader.GetAttribute("cogx")), Convert.ToDouble(reader.GetAttribute("cogy")), Convert.ToDouble(reader.GetAttribute("z")));
//Set the Axis of moment
myloadData.Aom = new Quaternion(Convert.ToDouble(reader.GetAttribute("aomx")), Convert.ToDouble(reader.GetAttribute("aomy")), Convert.ToDouble(reader.GetAttribute("aomz")), Convert.ToDouble(reader.GetAttribute("aomw")));
//The load can be considered a point mass, in this example, i.e. without any moment of inertia
myloadData.Inertia = new Vector3(0, 0, 0);
tool.LoadData = myloadData;
//Show the name of the tooldata in the graphics
tool.ShowName = true;
//Show the tool data in the graphics
tool.Visible = true;
//Add the tool data to the active task
station.ActiveTask.DataDeclarations.Add(tool);
[/code]
There are some problems I could not solve:
Part has a method called attach, but I have no clue how to use it
Is this possible with the API?
Best regards and a happy new year,
Matthias
I am trying to create a tool from a geometry.
There is an Xml-file with the data I need for the tool.
It stores the path to the Cad-file, its center of gravity, its position in the wrist coordinate system and so on.
I can import the geometry from a given CAD-model via
[code]
GraphicComponentLibrary gcl = GraphicComponentLibrary.Load(reader.GetAttribute("path"), false);
station.GraphicComponents.Add(gcl.RootComponent.CopyInstance ());
[/code]
I can also create a tool with data from an Xml-reader via
[code]
RsToolData tool = new RsToolData();
tool.ModuleName = "Module1";
tool.Name = reader.GetAttribute("name");
tool.RobotHold = true;
tool.Frame.X = Convert.ToDouble(reader.GetAttribute("x"));
tool.Frame.Y = Convert.ToDouble(reader.GetAttribute("y"));
tool.Frame.Z = Convert.ToDouble(reader.GetAttribute("z"));
tool.Frame.RX = Globals.DegToRad(Convert.ToDouble(reader.GetAttribute("rx")) );
tool.Frame.RY = Globals.DegToRad(Convert.ToDouble(reader.GetAttribute("ry")) );
tool.Frame.RZ = Globals.DegToRad(Convert.ToDouble(reader.GetAttribute("rz")) );
//Create the loaddata for the tool
RsLoadData myloadData = new RsLoadData();
//Set mass
myloadData.Mass = Convert.ToDouble(reader.GetAttribute("mass"));
//Set center of gravity
myloadData.Cog = new Vector3(Convert.ToDouble(reader.GetAttribute("cogx")), Convert.ToDouble(reader.GetAttribute("cogy")), Convert.ToDouble(reader.GetAttribute("z")));
//Set the Axis of moment
myloadData.Aom = new Quaternion(Convert.ToDouble(reader.GetAttribute("aomx")), Convert.ToDouble(reader.GetAttribute("aomy")), Convert.ToDouble(reader.GetAttribute("aomz")), Convert.ToDouble(reader.GetAttribute("aomw")));
//The load can be considered a point mass, in this example, i.e. without any moment of inertia
myloadData.Inertia = new Vector3(0, 0, 0);
tool.LoadData = myloadData;
//Show the name of the tooldata in the graphics
tool.ShowName = true;
//Show the tool data in the graphics
tool.Visible = true;
//Add the tool data to the active task
station.ActiveTask.DataDeclarations.Add(tool);
[/code]
There are some problems I could not solve:
- How can I attach the geometry to a robot? In Rs it is just drag&drop, but with the API...?!
- How can I connect the RsToolData with the geometry?
Part has a method called attach, but I have no clue how to use it

Is this possible with the API?
Best regards and a happy new year,
Matthias
0
Comments
-
Hi Matthias,
To attach a tool to a robot you can do like this:
actStn = Project.ActiveProject as Station;
Mechanism mechRobot = actStn.GraphicComponents["MyRobot"] as Mechanism;
GraphicComponent grTool = actStn.GraphicComponents["MyTool"];IAttachableChild attachTool = grTool as IAttachableChild;
mechRobot.GetFlanges()[0].Attach(attachTool, false);Best regards,
Anders Spaak
ABB Robotics0 -
Hi again!
Thanks for your help!
Now I have
[code]
GraphicComponentLibrary gcl = GraphicComponentLibrary.Load(reader.GetAttribute("path"), false);
GraphicComponent gc = gcl.RootComponent.CopyInstance();
gc.Name = reader.GetAttribute("name");
station.GraphicComponents.Add(gc);
Mechanism mechRobot = station.GraphicComponents["IRB4400_60_196__01_2"] as Mechanism;
GraphicComponent grTool = station.GraphicComponents[reader.GetAttribute("name")];
IAttachableChild attachTool = grTool as IAttachableChild;
mechRobot.GetFlanges()[0].Attach(attachTool, true);
[/code]
When I attach a tool to a robot manually in RS the TCP changes automatically.
Can I do this within a Plugin?
Best Regards, Matthias
Matthias2008-1-7 13:51:380 -
Hi,
when I use my plugin the tool is attached to the robot, but no new TCP is created.
When I detach the tool (right click on tool in Objects-window -> detach, without keeping position) and then re-attach it (right click on tool -> attach to, also without keeping current position) the right TCP is created.
How can this be? Is there any solution?
I guess I can specify the TCP by
[code]
IAttachableParent parent = mechRobot.GetFlanges()[0] as IAttachableParent;
parent.attach(attachtool, true, offset);
[/code]
If that is the solution, how can I get the offset? The offset is loaded when I import the *.rslib, but I don't know how to access it.
Best regards, Matthias
Matthias2008-1-9 11:2:560
Categories
- All Categories
- 5.5K RobotStudio
- 398 UpFeed
- 19 Tutorials
- 13 RobotApps
- 299 PowerPacs
- 405 RobotStudio S4
- 1.8K Developer Tools
- 250 ScreenMaker
- 2.8K Robot Controller
- 323 IRC5
- 63 OmniCore
- 7 RCS (Realistic Controller Simulation)
- 812 RAPID Programming
- 5 AppStudio
- 3 RobotStudio AR Viewer
- 19 Wizard Easy Programming
- 105 Collaborative Robots
- 5 Job listings