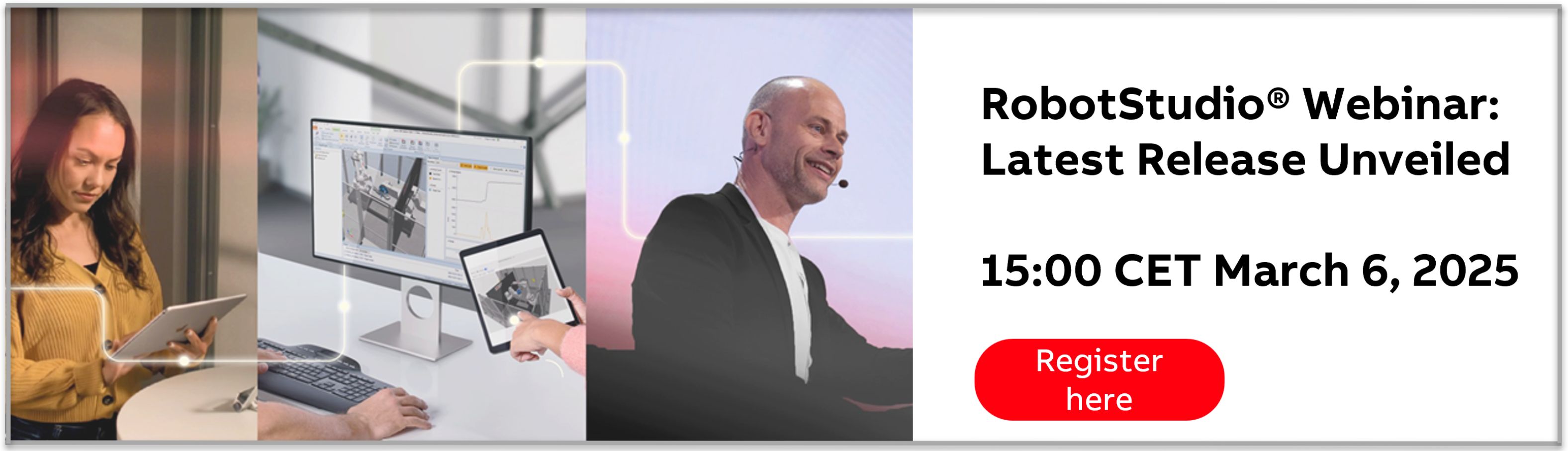
recursion and directory structure [WebWare SDK]
Comments
-
Hi Svempa,
i've written some routines to copy recursively some files and or directories, here they are:
'This function copies a directory structure including the files from
'a source to a destination drive and dir from a given robot.
'Also the structure can be deleted after copying from the source drive
'A user interface can be set up to hold status informations by handing
'over a textbox and a label.
Public Function S4DirCopy(Robot As Helper, _
SourceDrive As String, SourceDir As String, _
DestDrive, DestDir, _
DelAfterCopy As Boolean, Optional StatMessage As TextBox, _
Optional StatTitle As Label) As Integer
Dim DirList() As String
Dim NewDir As String
Dim NewDrive As String
Dim Sourcefile As String
Dim Destfile As String
Dim i As Integer
Dim ShortPath As String
Dim x As Integer
Dim dX As Integer
Dim hResult As Integer
On Local Error GoTo DirCopyHandler
'Setthe staus label text
StatTitle.Caption = "Status:"
'get a directory from the source dir
Result = Robot.S4Dir(SourceDrive, SourceDir, 0, WAIT_ON_RESULT, ResultID)
If Result = 0 Then
'Display status
StatMessage.Text = SourceDir & " read"
Else
S4DirCopy = Result
Exit Function
End If
'If subdirs found
If Robot.DirListCount > 0 Then
'Get storage place
ReDim DirList(Robot.DirListCount - 1)
End If
'If files found
If Robot.FileListCount > 0 Then
'Get storage place
ReDim FileList(Robot.FileListCount - 1)
End If
'For all found sub directories
For i = 0 To Robot.DirListCount - 1
'Copy to a local data storage string
DirList(i) = Robot.DirList(i)
Next
'Create destination dir (if it is the root then do not create it)
NewDir = DestDir
NewDrive = DestDrive
NewDir = RemoveDirSep(NewDir)
NewDrive = RemoveDirSep(NewDrive)
If NewDrive = S4C_Ramdisk Or NewDrive = S4CPlus_Ramdisk Then
If NewDir <> strEMPTY Then
Result = Robot.S4MkDir(DestDrive, DestDir, WAIT_ON_RESULT, ResultID)
End If
Else
If NewDir <> strEMPTY Then
Result = Robot.S4MkDir(DestDrive, DestDir, WAIT_ON_RESULT, ResultID)
End If
End If
'Set status info
StatMessage.Text = DestDrive & DestDir & " created"
'for all Files do
For i = 0 To Robot.FileListCount - 1
'Get filenames
Sourcefile = AddDirSep(SourceDir, True) & Robot.FileList(i)
Destfile = DestDir
Destfile = AddDirSep(Destfile, True)
Destfile = Destfile & Robot.FileList(i)
'Set status info
StatTitle.Caption = "Copying"
StatMessage.Text = Sourcefile
'Copy the file
Result = Robot.S4FileCopy(SourceDrive, Sourcefile, DestDrive, Destfile, _
WAIT_ON_RESULT, ResultID)
S4DirCopy = Result
If Result = 0 Then
'If delete after copy then delete the file
If DelAfterCopy = True Then
'Set status info
StatTitle.Caption = "Deleting "
StatMessage.Text = Sourcefile
'delete file
Result = Robot.S4FileDelete(SourceDrive, Sourcefile, WAIT_ON_RESULT, ResultID)
S4DirCopy = Result
End If
Else
'give error during copy
MsgBox "Couldn't copy the file, error code = " & Result & "."
End If
Next
'For all sub directories
For i = Robot.DirListCount - 1 To 0 Step -1
'Exclude parent and actual directory
If DirList(i) <> "." And DirList(i) <> ".." Then
'Call S4DirCopy recursively with next directory
Result = S4DirCopy(Robot, SourceDrive, AddDirSep(SourceDir, True) & DirList(i), _
DestDrive, DestDir & "/" & DirList(i), _
DelAfterCopy, StatMessage, StatTitle)
If Result = 0 Then
'If delete after copy ordered
If DelAfterCopy = True Then
'Set status info
StatTitle.Caption = "Deleting directory"
StatMessage.Text = DirList(i)
'Delete directory from source drive
Result = Robot.S4RmDir(SourceDrive, AddDirSep(SourceDir, True) & DirList(i), _
WAIT_ON_RESULT, ResultID)
S4DirCopy = Result
End If
End If
End If
Next
'If delete after copy ordered
If DelAfterCopy = True Then
'Set status info
StatTitle.Caption = "Deleting directory"
StatMessage.Text = SourceDir
'Delete directory from source drive
Result = Robot.S4RmDir(SourceDrive, SourceDir, WAIT_ON_RESULT, ResultID)
S4DirCopy = Result
End If
Exit FunctionDirCopyHandler:
If Err = 7 Then 'If Out of Memory error occurs, assume the list box just got full.
S4DirCopy = True 'Create Msg and set return value AbandonSearch.
Exit Function 'Note that the exit procedure resets Err to 0.
Else 'Otherwise display error message and quit.
Exit Function
End If
End FunctionI have also done a routine just for deleting this stuff:
'Function S4DirDelete deletes a directory
0 -
any code for the AddDirSep(), RemoveDirSep() ? I found some in VB forums, but they do not seem to match with their params.0
-
Hi sarnold688,please find here the code of those two routines.RegardsThomas'-----------------------------------------------------------
' SUB: AddDirSep
' Add a trailing directory path separator (slash or back slash) to the
' end of a pathname unless one already exists
'
' IN/OUT: [strPathName] - path to add separator to' Optional IN: [Forward] - switch to indicate which slash is to be added
'-----------------------------------------------------------
'
Public Function AddDirSep(strPathName As String, Optional Forward As Boolean = False) As String
Dim gstrSEP_URLDIR As String
Dim gstrSEP_DIR As StringgstrSEP_URLDIR = "/"
gstrSEP_DIR = ""
strPathName = RTrim$(strPathName)' Check if at the end the / is found
If Right$(strPathName, Len(gstrSEP_URLDIR)) <> gstrSEP_URLDIR Then
' Check if at the end a is foundIf Right$(strPathName, Len(gstrSEP_DIR)) <> gstrSEP_DIR Then
' If no separator found add the right one depending on flag ForwardIf Forward = True Then
strPathName = strPathName & gstrSEP_URLDIR
Else
strPathName = strPathName & gstrSEP_DIR
End If
End If
End If' Return resulting string
AddDirSep = strPathName
End Function'RemoveDirSep removes a trailing / or
Public Function RemoveDirSep(strPath As String) As String
Dim gstrSEP_URLDIR As String
Dim gstrSEP_DIR As StringgstrSEP_URLDIR = "/"
gstrSEP_DIR = ""
strPath = RTrim$(strPath)' if one of the separators found
If Right$(strPath, 1) = gstrSEP_DIR Or Right$(strPath, 1) = gstrSEP_URLDIR Then
' remove itstrPath = Mid(strPath, 1, Len(strPath) - 1)
End If' return result
RemoveDirSep = strPath
End Function0
Categories
- All Categories
- 5.6K RobotStudio
- 398 UpFeed
- 19 Tutorials
- 13 RobotApps
- 299 PowerPacs
- 405 RobotStudio S4
- 1.8K Developer Tools
- 250 ScreenMaker
- 2.8K Robot Controller
- 327 IRC5
- 64 OmniCore
- 7 RCS (Realistic Controller Simulation)
- 820 RAPID Programming
- 6 AppStudio
- 3 RobotStudio AR Viewer
- 19 Wizard Easy Programming
- 105 Collaborative Robots
- 5 Job listings