How can I pull a variable from the module/routine with pc sdk?
Options
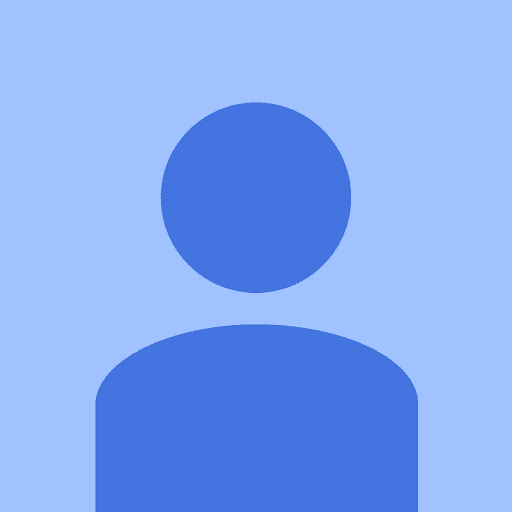
EugeneB
✭
I set up a variable in my module - var time. How can I pull this variable info from that module/routine with pc sdk?
0
Best Answer
Answers
-
This should do what you want, it will return all rob targets for each task.
RsTaskCollection tasks = irc5controller.Tasks; foreach (RsTask task in tasks) { RsDataDeclaration[] datadecls = task.FindDataDeclarationsByType(typeof(RsRobTarget)); }
You just need to change the RsRobTarget for what ever data type your variable is.0 -
RsTaskCollection is ABB.Robotics.RobotStudio.Stations class and irc5controller is an instance of the Controller class correct? It given me an error in this line: RsTaskCollection tasks = irc5controller.Tasks - problem with Tasks - "irc5controller" does not contain a definition for the Tasks. How can I do this without of using RobotStudio classes?0
-
Here what I've post to EugeneB for other:You can found in GetModule() how I do to find data.
//First prepare search<br> RapidSymbolSearchProperties rssp = RapidSymbolSearchProperties.CreateDefault();<br> rssp.GlobalSymbols = true;<br> rssp.InUse = false;<br> rssp.LocalSymbols = true;<br> rssp.Recursive = false;<br> rssp.SearchMethod = SymbolSearchMethod.Block;<br> rssp.Types = SymbolTypes.Data;<br>//Then look at all data in module<br> string typeName = "";// "GfxShapeData"; Don't find GfxShapeData Directly.<br> RapidSymbol[] rsArray = module.SearchRapidSymbol(rssp, typeName, string.Empty);<br> foreach (RapidSymbol rs in rsArray)<br> {<br> RapidData rd = module.GetRapidData(rs);<br> RapidDataType rdt = controller.Rapid.GetRapidDataType(rs);//Only grabe GfxShapeData, here you can do what you want like read rd.Name if (rd.RapidType == "GfxShapeData")<br> {<br> sDatasList += ";" + rs.Name;<br> }<br> }
In GetGfxShapeData I pull data, it's more complicated as it's a record type. RapidData rd = module.GetRapidData(sDataName);<br> if (rd.RapidType == "GfxShapeData")<br> {<br> RapidDataType rdt = controller.Rapid.GetRapidDataType(task.Name, module.Name, rd.Name);<br><br> sd = new GfxShapeData(rdt, rd);<br> if (sd.IsNotNull)<br> {<br> //Update Properties<br> component.Properties["ShapeType"].Value = (sd.ShapeType == GfxShapeData.ShapeTypes.Box) ? "Box" : "Capsule";<br> component.Properties["ParentAxis"].Value = sd.ParentAxis.Value;<br>
Here a simple example to get a PERS bool bTest:=FALSE; value: RapidData test = module.GetRapidData("bTest");<br> string s = test.StringValue;<br> Bool bTest = (Bool)test.Value;
Bool is on namespace ABB.Robotics.Controllers.RapidDomain.
☑️2024 - RobotStudio® User Group0
Categories
- All Categories
- 5.6K RobotStudio
- 399 UpFeed
- 20 Tutorials
- 14 RobotApps
- 301 PowerPacs
- 406 RobotStudio S4
- 1.8K Developer Tools
- 250 ScreenMaker
- 2.8K Robot Controller
- 345 IRC5
- 73 OmniCore
- 8 RCS (Realistic Controller Simulation)
- 844 RAPID Programming
- 20 AppStudio
- 4 RobotStudio AR Viewer
- 19 Wizard Easy Programming
- 107 Collaborative Robots
- 5 Job listings