Is it possible to parse file from end to beginning?
Options
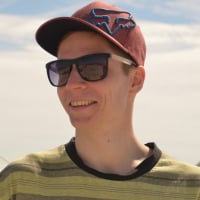
egor
✭
I can read and parse txt file from the beginning to the end this way:
Open "HOME:/Test.txt",file\Read;
Rewind file;
WHILE EOF=TRUE DO
Read_Str:=ReadStr(file);
ENDWHILE
But how to read the file in reverse order? Is it possible using "ReadStr" function?
0
Answers
-
You could probably count the lines of the file with a FOR loop from beginning until EOF, then use ReadStr with \Line to start with the last line and FOR loop from the last line to the first line.0
-
Seemed to work -- as long as the line is not too long and can fit into a single string...PROC exampleReadFileInReverse()VAR string text;
VAR iodev infile;
VAR num lineCounter:=0;
Open "HOME:/test.txt",infile\Read;
! count number of lines in the file
lineCounter:=0;
WHILE text <> EOF DO
IF text <> EOF THEN
text := ReadStr(infile\Line:=lineCounter+1);
IF text <> EOF lineCounter:=lineCounter+1;
ENDIF
ENDWHILE
TPWrite "File has "+NumToStr(lineCounter,0)+" lines.";
! read file in reverse
FOR i FROM lineCounter TO 1 STEP -1 DO
text := ReadStr(infile\Line:=i);
TPWrite "Line #"+NumToStr(i,0)+" = "+text;
WaitTime 1;
ENDFOR
ENDPROC
2 -
soup said:Seemed to work -- as long as the line is not too long and can fit into a single string...0
-
Looks good.To clarify: I was stating that you'd have a problem if a single line is long, rather than a large quantity of lines -- make a test line with a hundred chars to see what I mean.0
Categories
- All Categories
- 5.6K RobotStudio
- 400 UpFeed
- 20 Tutorials
- 14 RobotApps
- 301 PowerPacs
- 406 RobotStudio S4
- 1.8K Developer Tools
- 250 ScreenMaker
- 2.8K Robot Controller
- 346 IRC5
- 73 OmniCore
- 8 RCS (Realistic Controller Simulation)
- 844 RAPID Programming
- 20 AppStudio
- 4 RobotStudio AR Viewer
- 19 Wizard Easy Programming
- 108 Collaborative Robots
- 5 Job listings